문제설명
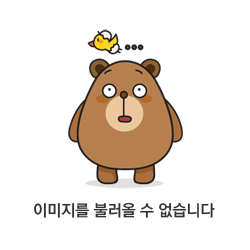
입출력 예제
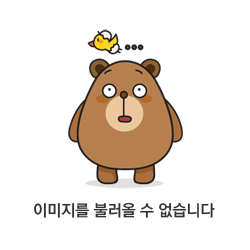
개념
단어를 길이를 기준으로 정렬하는 문제다. std::sort에 비교 구문을 추가하여 해결하면 쉽게 해결할 수 있다.
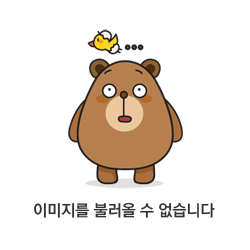
풀이
#include <iostream>
#include <vector>
#include <algorithm>
int main()
{
int N;
std::cin >> N;
std::vector<std::string> v;
while (N--)
{
std::string str;
std::cin >> str;
v.push_back(str);
}
단어의 개수 N을 초기화하고, 문자열을 넣을 벡터 v를 초기화해 준다.
struct
{
bool operator() (std::string a, std::string b) const
{
if (a.size() == b.size())
return a < b;
return (a.size() < b.size());
}
} custom;
std::sort(v.begin(), v.end(), custom);
v.erase(std::unique(v.begin(), v.end()), v.end());
for (std::string str : v)
std::cout << str << '\n';
}
std::sort에 추가할 비교문 custom을 구조체를 이용해 구현한다. 만약 길이가 같은 경우라면, 사전 순으로 정렬하게 하고, 다른 경우엔 길이가 짧은 것이 앞으로 오게 해 준다.
이후엔 erase() 함수와 unique() 함수를 통해 중복되는 것을 지워주고, 범위 기반 for문을 통해 벡터에 있는 원소를 출력하면 된다.
총합본
#include <iostream>
#include <vector>
#include <algorithm>
int main()
{
int N;
std::cin >> N;
std::vector<std::string> v;
while (N--)
{
std::string str;
std::cin >> str;
v.push_back(str);
}
struct
{
bool operator() (std::string a, std::string b) const
{
if (a.size() == b.size())
return a < b;
return (a.size() < b.size());
}
} custom;
std::sort(v.begin(), v.end(), custom);
v.erase(std::unique(v.begin(), v.end()), v.end());
for (std::string str : v)
std::cout << str << '\n';
}
반응형
'Algorithm > 백준' 카테고리의 다른 글
[백준] 18870 좌표 압축 with C++ (0) | 2023.04.15 |
---|---|
[백준] 10814 나이순 정렬 with C++ (0) | 2023.04.14 |
[백준] 11650 with C++ (0) | 2023.04.12 |
[백준] 1427 with C++ (0) | 2023.04.11 |
[백준] 2751 with C++ (0) | 2023.04.10 |