문제 설명
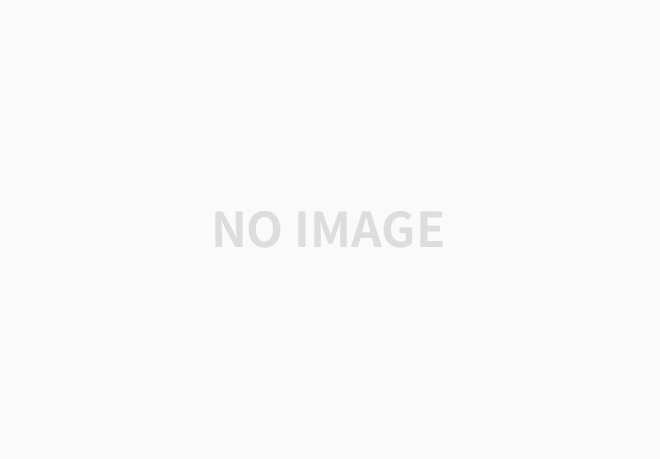
제한 사항 및 입출력 예제
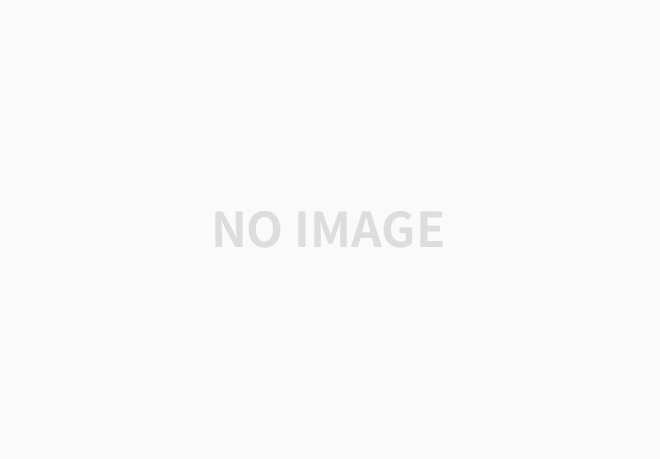
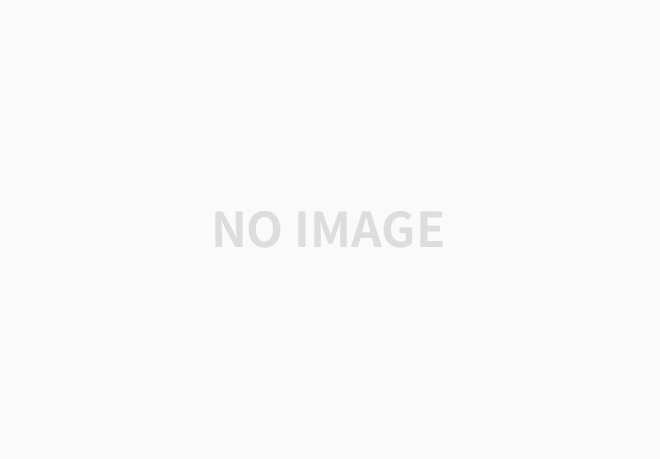
개념
개인정보의 수집 유효기간을 확인하는 문제다. 이 문제에서는 오늘 날짜와 각 개인정보의 수집일을 바탕으로 개인정보의 수집이 유효한지, 아니면 파기해야 하는지를 판단하게 된다. 이때, 날짜는 YYYY.MM.DD 형태로, 개인정보는 YYYY.MM.DD A 형태로 주어집니다.
각 개인정보에는 수집일과 개인정보 유형(A)이 포함되어 있다. 이 유형은 유효 기간을 결정한다. 예를 들어, A 유형의 개인정보는 수집일로부터 특정 기간 동안만 유효한다. 유효 기간은 'terms'라는 문자열 벡터에 정의되어 있으며, 각 문자열은 유형과 그 유형의 유효 기간을 나타내는 월 단위의 숫자로 구성되어 있다.
따라서 이 문제의 목표는 오늘 날짜를 기준으로 개인정보의 유효 기간이 지났는지를 판별하는 것이다. 유효 기간이 지난 개인정보의 경우 해당 정보의 인덱스를 결과 벡터에 추가하면 된다. 이 과정을 통해 문자열 처리 및 날짜 비교 기법을 활용하게 된다.
풀이
#include <vector>
#include <string>
#include <map>
using namespace std;
vector<int> solution(string today, vector<string> terms, vector<string> privacies)
{
vector<int> answer;
map<char, int> m;
int year, month, day, std_month;
for (int i = 0; i < terms.size(); ++i)
m[terms[i][0]] = stoi(terms[i].substr(2));
오늘과 약관 기간을 비교하기 위해 year, month, day, std_month를 초기화하고, 개인정보 유형을 맵에 저장한다.
for (int i = 0; i < privacies.size(); ++i)
{
year = (stoi(today.substr(0, 4)) - stoi(privacies[i].substr(0, 4))) * 12;
month = stoi(today.substr(5, 2)) - stoi(privacies[i].substr(5, 2));
day = stoi(today.substr(8,2)) - stoi(privacies[i].substr(8, 2));
std_month = m[privacies[i][11]];
오늘과 개인정보의 수집일 사이의 시간을 년, 월, 일 단위로 계산하고, 개인정보의 유형에 따른 유효기간을 찾아 std_month에 저장한다.
if (std_month < year + month)
answer.push_back(i + 1);
else if (std_month == year + month && day >= 0)
answer.push_back(i + 1);
}
return answer;
}
유효 기간이 지났는지 검사하고, 지났으면 해당 개인정보의 인덱스를 추가하고, 만약 유형의 유효 기간이 지나지 않았거나 같은데 일자가 지나지 않았을 경우는 제외한다.
총합본
#include <vector>
#include <string>
#include <map>
using namespace std;
vector<int> solution(string today, vector<string> terms, vector<string> privacies)
{
vector<int> answer;
map<char, int> m;
int year, month, day, std_month;
for (int i = 0; i < terms.size(); ++i)
m[terms[i][0]] = stoi(terms[i].substr(2));
for (int i = 0; i < privacies.size(); ++i)
{
year = (stoi(today.substr(0, 4)) - stoi(privacies[i].substr(0, 4))) * 12;
month = stoi(today.substr(5, 2)) - stoi(privacies[i].substr(5, 2));
day = stoi(today.substr(8,2)) - stoi(privacies[i].substr(8, 2));
std_month = m[privacies[i][11]];
if (std_month < year + month)
answer.push_back(i + 1);
else if (std_month == year + month && day >= 0)
answer.push_back(i + 1);
}
return answer;
}
'Algorithm > 프로그래머스' 카테고리의 다른 글
[프로그래머스] 신규 아이디 with C++ (0) | 2023.08.24 |
---|---|
[프로그래머스] 귤 고르기 with C++ (0) | 2023.06.28 |
[프로그래머스] 소수 찾기 with C++ (0) | 2023.06.19 |
[프로그래머스] 행렬의 곱셈 with C++ (2) | 2023.06.18 |
[프로그래머스] N개의 최소공배수 with C++ (0) | 2023.06.13 |